class: middle # Removing fragility from software with the **EYG** programming language --- class: middle ## **Hi** ### *name -* Peter Saxton ### *@internets -* CrowdHailer ### *works -* Experimenting in building better languages and tools at [eyg.run](https://eyg.run)
--- class: middle ## What is **fragility**? How susceptible is something to damage or breaking? --- class: middle ## Why do programs **break**? You probably have an intuition. --- class: middle ### ChatGPT 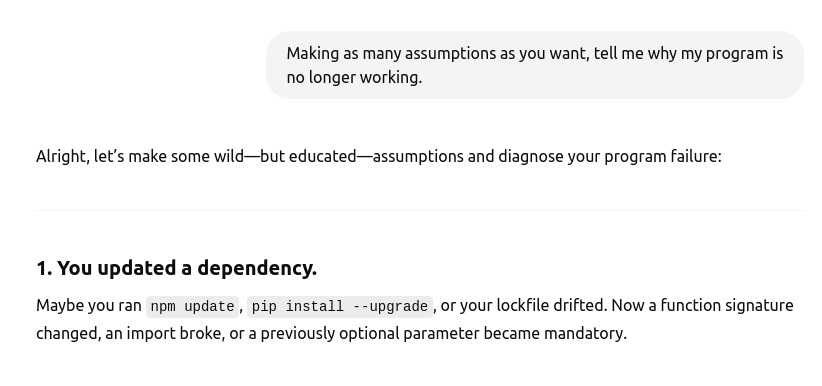 --- class: middle 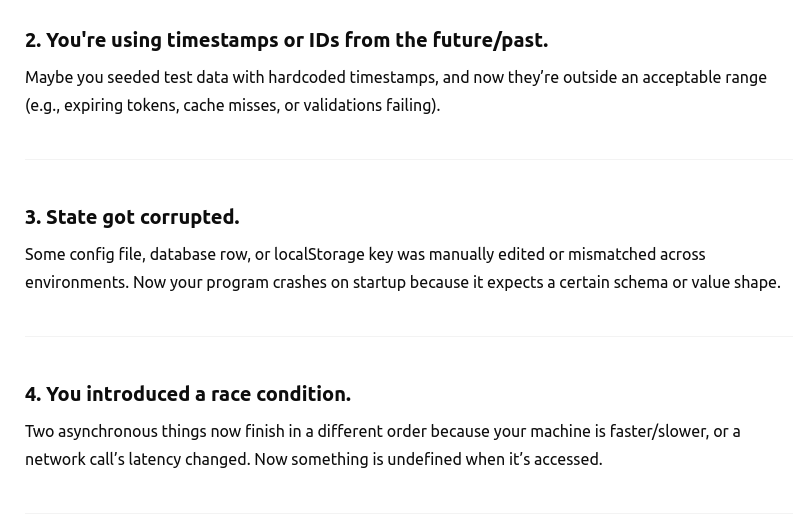 --- class: middle 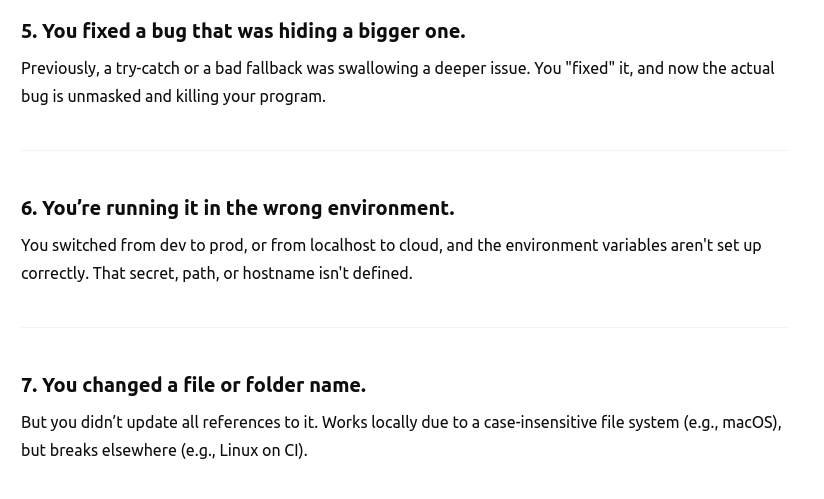 --- class: middle 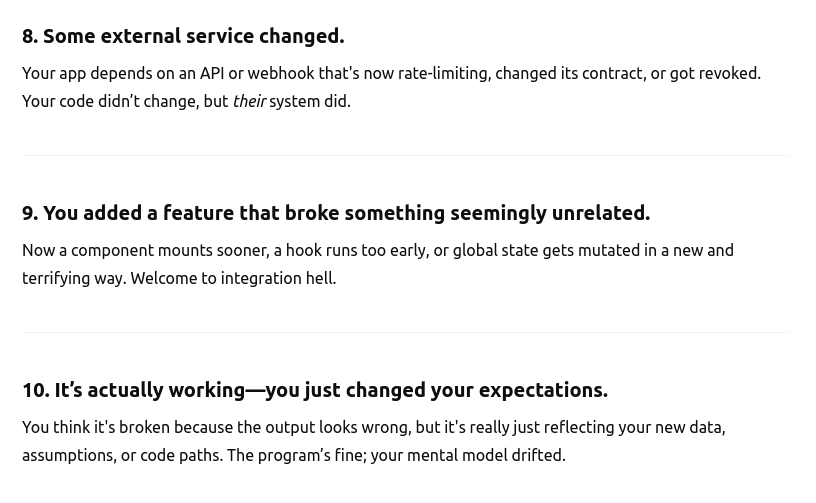 --- class: middle ## What helps - Type systems - Formal proofs - Simulation testing - Immutability - Containers --- class: middle ### Not crashing is **enough** 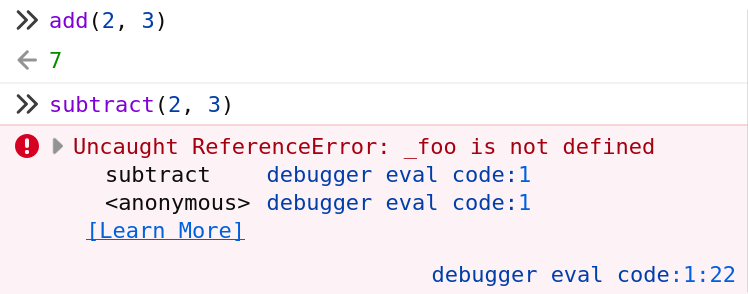 --- class: middle ## Where do **crashes** come from? --- class: middle ## Crashes are **Weird** - Lambda calculus cannot crash - Lambda calculus may not terminate - `(λx. x x) (λx. x x)` - We added the ability to crash. --- class: middle ```py import sys data = sys.stdin.read() print("You entered:") print(data) ``` ```sh EOFError: EOF when reading a line ``` --- class: middle ## What is **EYG?** A way to write programs that: - Never crash. - Run anywhere. Make any invalid state unrepresentable --- class: middle ## EYG the **Basics** - Statically typed - Structurally typed - Sound type inference - Algebraic effects - Closure serialization - Strongly typed REPL - Inline references - Minimal AST --- class: middle
--- class: middle ## What is **Eat Your Greens**? > Beyond diet, "eat your greens" can symbolize doing things that are good for you, even if they're not immediately enjoyable or easy. --- class: middle # Features of **EYG** - Algebraic effects - Structural typing - Hashed dependencies --- class: middle ## Effects 1. **What can they do?** 2. How do they run? --- class: middle ## **Algebraic** effects An abstraction to represent all impure behaviour including: - Input and output operations - Non determinism i.e. random - Time - Exceptions - Concurrency - Mutability --- class: middle
**Demo time:** using effects --- class: middle ### **Algebraic** effects Represent every interaction with the real world in the type system. ``` add: (Int, Int) -> Int alert: (String
) -> {} map: (List(0), (0 <..2>) -> 1 <..2>) -> List(1) ``` Typing of effects is **sound** and **extensible**. --- class: middle
**Demo time:** typing effects --- class: middle ### **Algebraic** effects Effects become the only interface Effects are an interesting design space for their impact on developer experience. ``` tweet: (String
) -> {} tweet: (String
) -> {} tweet: (String
) -> {} ``` The platform can offer anything as an effect. ``` is_cat: (Binary
) -> True {} | False {} ``` --- class: middle ### **Algebraic** effects Platforms can offer any subset of effects for programs to run against.  [**erlang recently got tty*](https://www.erlang.org/news/174#shell-and-terminal) --- class: middle ### **Algebraic** effects Effects are a uniform interface - Singular approach for mocking in tests - A basic permissions system --- class: middle
**Demo time:** handling effects --- class: middle ## Effects 1. What they can do? 2. **How do they run?** --- class: middle ### What is a **function**? > A function is the relation between a set of inputs and a set of outputs, so that every set of inputs has exactly one output. 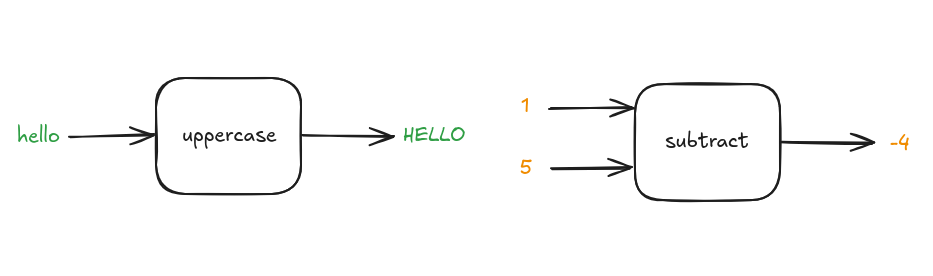 --- class: middle Functions compose. 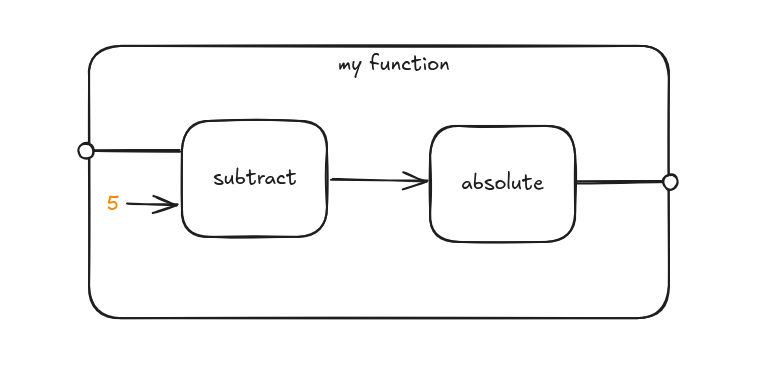 Functions can be values. 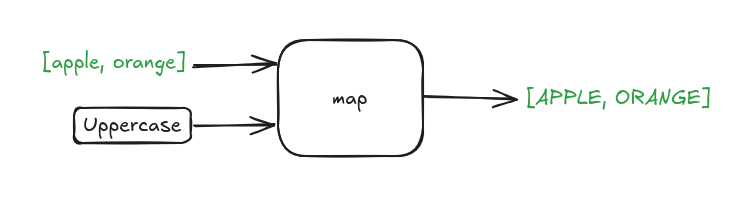 --- class: middle ### Side **effects** and side **causes** 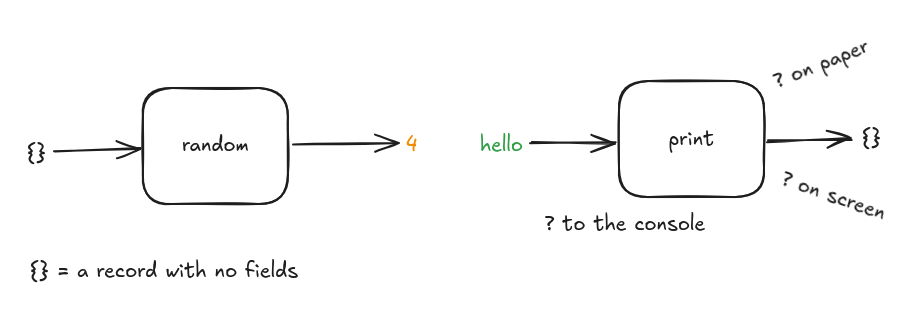 -- 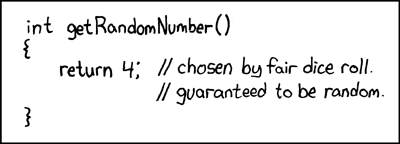 --- class: middle Side effects depend on the whole world. 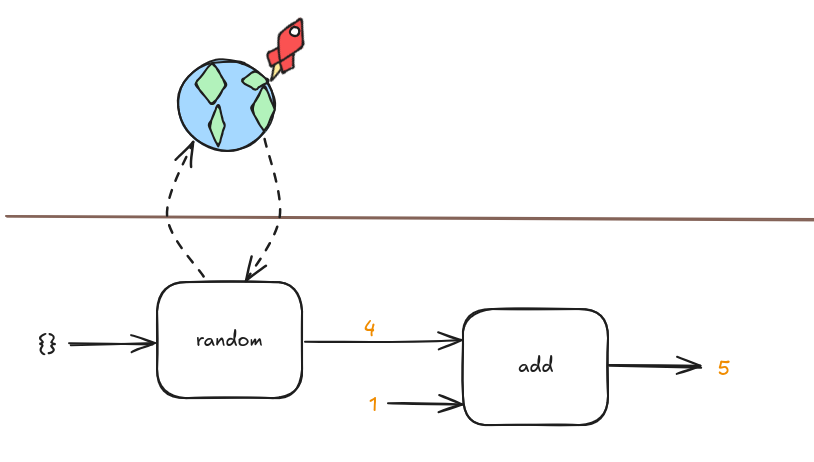 --- class: middle A quick aside for **continuations** 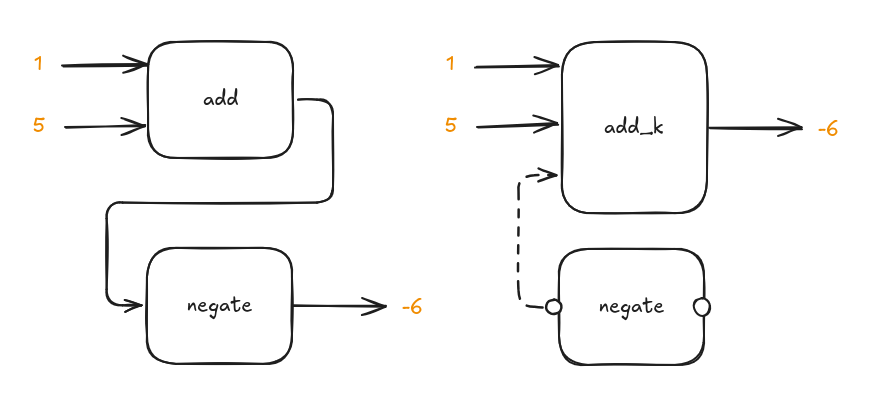 *The best resource explaining continuations is. [Delimited Continuations, Demystified by Alexis King](https://www.youtube.com/watch?v=TE48LsgVlIU)* --- class: middle Programs **perform** effects with the remaining program captured as a continuation. 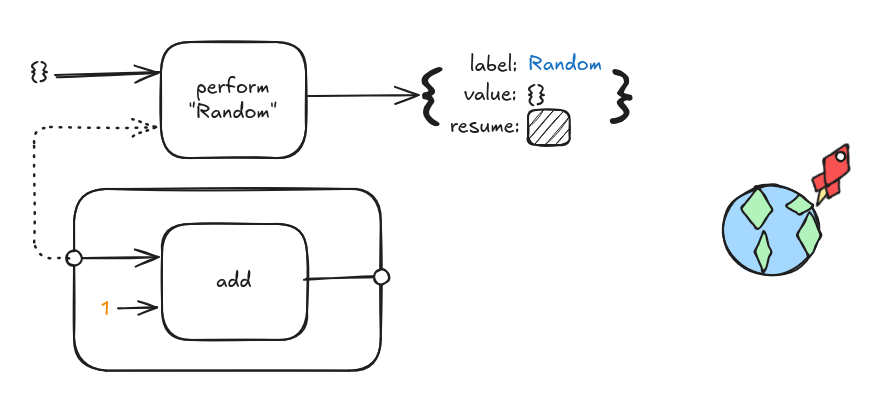 --- class: middle Runtimes **resume** programs by calling the stored continuation 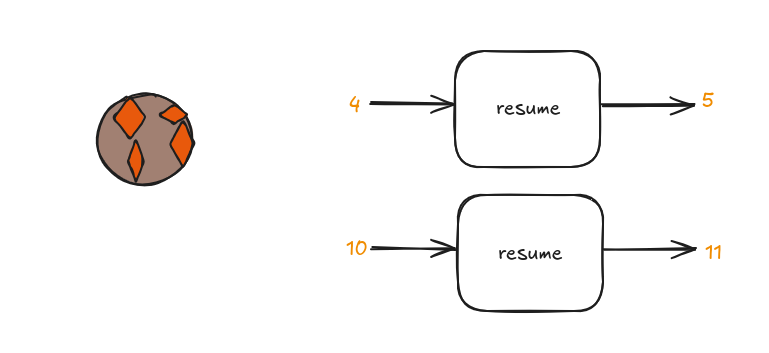 --- class: middle ## **Never** crash? - An EYG program is deterministic in the absence of effects. - EYG programs are serializable - A serialized continuation can always be run on another computer with the same input. --- class: middle # Structural typing --- class: middle ### Benefits of a type system 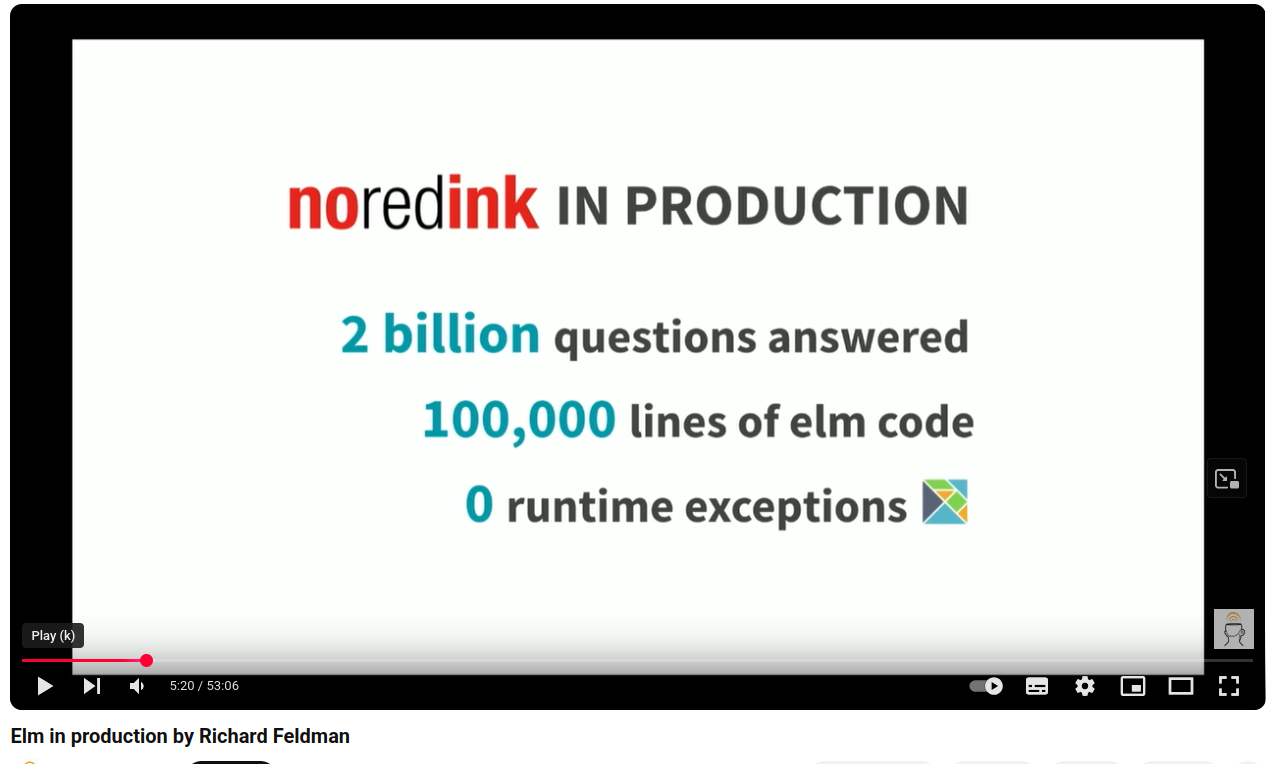 --- class: middle ### Benefits of a type system What do types add to this Python program? ```py print("Hello, World!") ``` Did you appreciate types the first day you were coding? --- class: middle What does a **type system** offer. - Consistency based on some axioms. - The axioms are the perimeter. - The wider the perimeter of our axioms the more valuable the type system. --- class: middle ### Web development 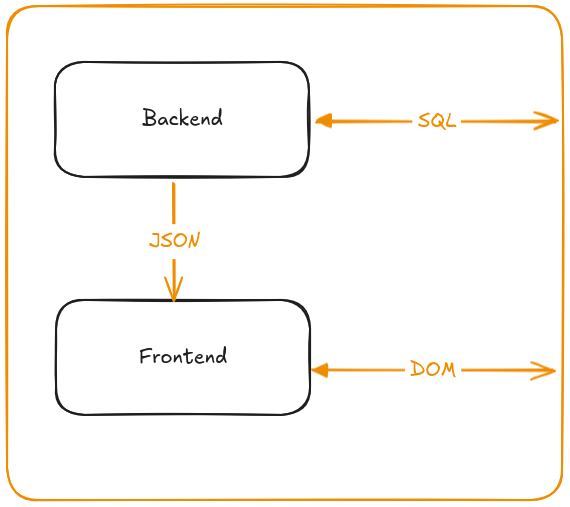 --- class: middle ### Metaframeworks, Liveview 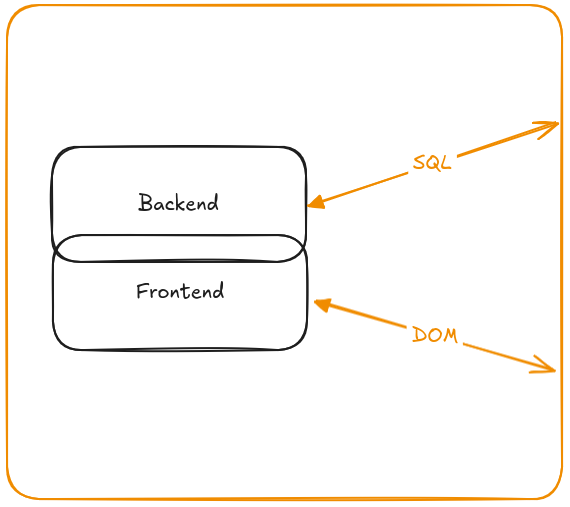 --- class: middle ### Web development It's far more than a frontend/backend split - Local development - Build servers - CI/CD - codegen - Backend/frontend - Service workers - Background queues - Infra provisioning - Database queries How often do we statically check the glue? --- class: middle 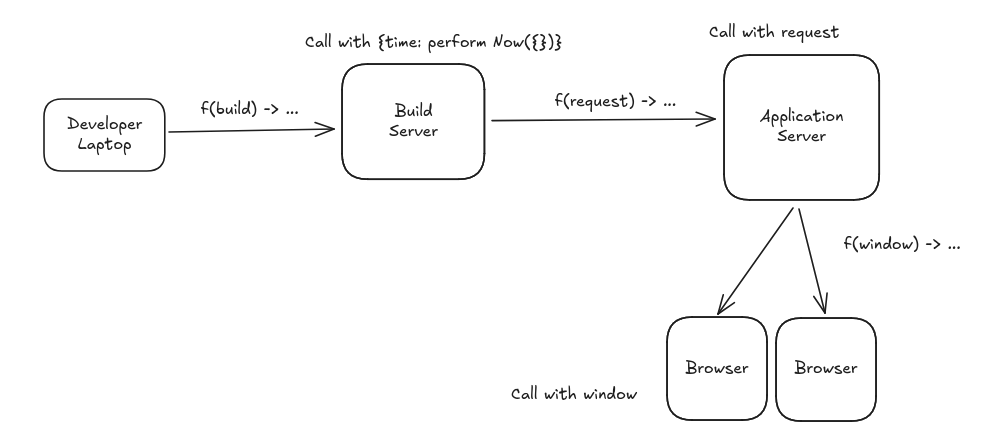 --- class: middle ### Closure serialization --- class: middle ### Closure serialization --- class: middle ### Thought experiment Imagine a **perfect** language, but: - libc is not installed - You're calling an undocumented JSON API - You need to implement OAuth to get a token - The deployment pipeline is a lot of bash --- class: middle ### Unix philosophy 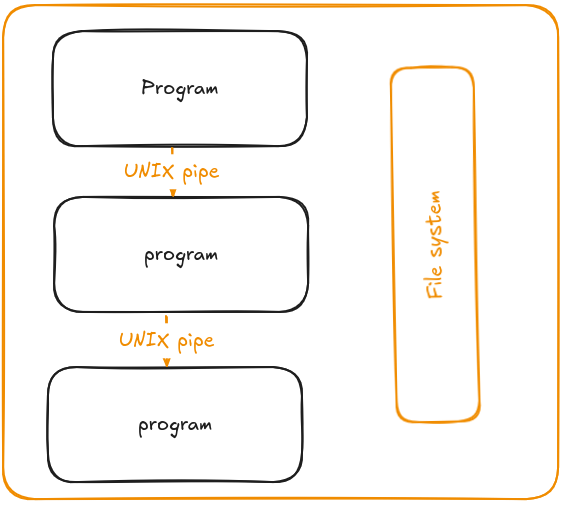 --- class: middle ### Kubernetes 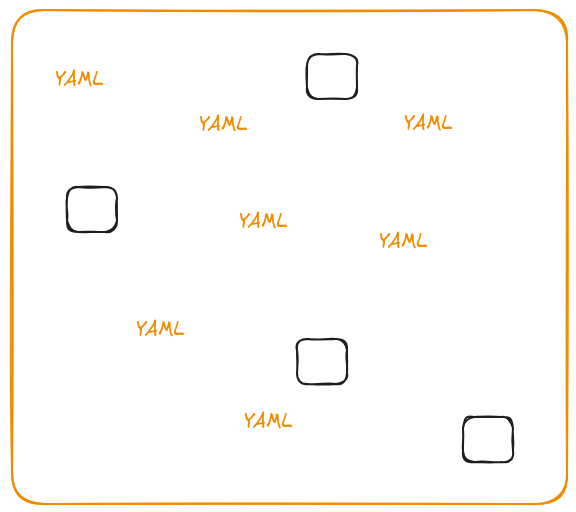 --- class: middle ### Can we type check the **system**? yes --- class: middle ### Structural or Nominal typing? - Structural types are **universal** - Nominal types have a bounded validity, *usually a single compilation.* - Nominal typing requires type declarations --- class: middle ### Structural or Nominal typing? Java ```java class Person { public name: string; } ``` TypeScript ```ts interface Person { name: string; } ``` --- class: middle ### Deployment 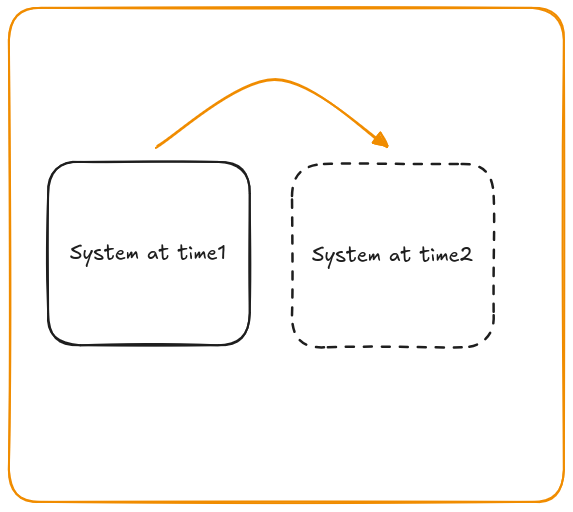 --- class: middle Demo code reloading --- class: middle ### Deterministic dependencies - Every dependency has an inline checksum - Every dependency is pure and it's type is narrowed. --- class: middle
--- class: middle ## Eat your **Greens** A simple type system leveraged everywhere is more interesting than a complex one limited to the application. - Development environments - Build servers - CI/CD - codegen - Backend/frontend - Service workers - Background queues - Infra provisioning - Database queries A single typed program should extend over the whole stack --- class: middle ## Run anywhere It's all about the effects. - Transpile to Google Apps script - Transpile to Figma Plugin - Transpile to tinyGo --- class: middle ## Thank you https://eyg.run - https://eyg.run/news - https://eyg.run/roadmap